ECMAScript Built-in Constants and Functions About Mathematics
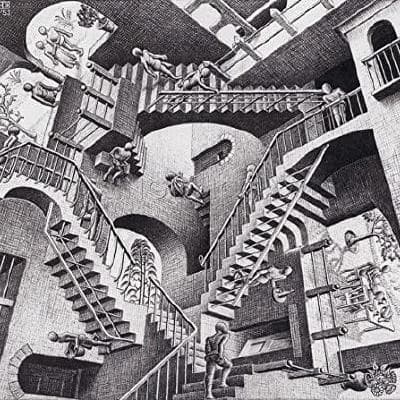
Shen Lu
Posted on Feb 08, 2024
views views
8 min read (1433 words)
ECMAScript Global Object for Mathematics
In ECMAScript, there are plenty of built-in objects that can be directly used as mathematical constants and functions.
Value Properties
Value Properties | Description | Notation | Value (Number) |
---|---|---|---|
Infinity | positive Infinity | Infinity (+Infinity) | |
NaN | Not a Number | NaN |
Function Properties
Function Properties | Description | Return Type |
---|---|---|
isFinite(number) | determine whether a number is a finite number or not (Number.isFinite(Number(number)) ) | boolean |
isNaN(number) | determine whether a value is NaN or not (Number(number) !== Number(number) ) | boolean |
parseFloat(string) | parse an argument to a floating point number | number (float) |
parseInt(string, radix) | parse an argument to an integer of the specified radix | number (integer) |
Constructor Properties
- BigInt: A built-in object whose constructor returns a bigint primitive (or BigInt)to represent whole numbers larger than (
Number.MAX_SAFE_INTEGER
), which is the largest number JavaScript can represent with a number primitive (or Number value). - Numbers: A primitive wrapper object used to represent and manipulate numbers and its type is a double-precision 64-bit binary format IEEE 754
BigInt
Function Properties | Description | Return Type |
---|---|---|
asIntN(bits, bigint) | clamp a BigInt value to a signed integer value | bigint |
asUintN(bits, bigint) | clamp a BigInt value to an unsigned integer value | bigint |
Numbers
Value Properties | Description | Notation | Value (Number) |
---|---|---|---|
EPSILON | the magnitude of the difference between 1 and the smallest value greater than 1, which is approximately | 2.220446049250313e-16 | |
NaN | the value identicial to the global NaN | NaN | |
MAX_SAFE_INTEGER | the maximum safe integer in JavaScript | 9007199254740991 | |
MAX_VALUE | the largest positive representable number | 1.7976931348623157e+308 | |
MIN_SAFE_INTEGER | the minimum safe integer in JavaScript () | -9007199254740991 | |
MIN_VALUE | the smallest positive representable number—that is, the positive number closest to zero (without actually being zero) | 5e-324 | |
NEGATIVE_INFINITY | negative infinity | -Infinity | |
POSITIVE_INFINITY | the value identicial to the global Infinity | Infinity (+Infinity) |
Function Properties | Description | Return Type |
---|---|---|
isFinite(number) | determine whether the passed value is a finite number or not | boolean |
isInteger(number) | determine whether the passed value is an integer or not | boolean |
isNaN(number) | determine whether the passed value is NaN or not (typeof number === 'number' && number !== number ) | boolean |
isSafeInteger(number) | determine whether the passed value is a safe integer (between and ) or not | boolean |
parseFloat(string) | the function identicial to the global parseFloat | number (float) |
parseInt(string, radix) | the function identicial to the global parseInt | number (integer) |
Note:
Number.isFinite(number)
does not convert its argument to a Number before determining whether it isInfinity
or not, which differs from the globalisFinite(number)
function. SoNumber.isNaN(number)
does it as well.
Other Properties
- Math: A a built-in object that has properties and methods for mathematical constants and functions. It’s not a function object.
Math
works with theNumber
type. It doesn't work withBigInt
.
Math
Value Properties | Description | Notation | Value (Number) |
---|---|---|---|
E | the base of the natural logarithms | 2.7182818284590452354 | |
LN10 | the natural logarithm of 10 | 2.302585092994046 | |
LN2 | the natural logarithm of 2 | 0.6931471805599453 | |
LOG10E | the base-10 logarithm of | 0.4342944819032518 | |
LOG2E | the base-2 logarithm of | 1.4426950408889634 | |
PI | the ratio of the circumference of a circle to its diameter | 3.1415926535897932 | |
SQRT1_2 | the square root of ½ | 0.7071067811865476 | |
SQRT2 | the square root of 2 | 1.4142135623730951 |
Function Properties | Description | Notation | Return Type |
---|---|---|---|
abs(x) | return the absolute value of x | number | |
acos(x) | return the inverse cosine of x | number | |
acosh(x) | return the inverse hyperbolic cosine of x | number | |
asin(x) | return the inverse sine of x | number | |
asinh(x) | return the inverse hyperbolic sine of x | number | |
atan(x) | return the inverse tangent of x | number | |
atanh(x) | return the inverse hyperbolic tangent of x | number | |
atan2(y, x) | return the inverse tangent of the quotient y / x of the arguments y and x | number | |
cbrt(x) | return the cube root of x | number | |
ceil(x) | return the smallest integer greater than or equal to x | number | |
clz32(x) | return the number of leading zero bits of the 32-bit integer x | number | |
cos(x) | return the cosine of x | number | |
cosh(x) | return the hyperbolic cosine of x | number | |
exp(x) | return the exponential function of x | number | |
expm1(x) | return the result of subtracting 1 from the exponential function of x | number | |
floor(x) | return the largest integer less than or equal to x | number | |
fround(x) | return the nearest single precision float representation of x | number | |
hypot(...args) | return the square root of the sum of squares of its arguments. | number | |
imul(x, y) | return the result of the 32-bit integer multiplication of x and y. | number | |
log(x) | return the natural logarithm of x | number | |
log1p(x) | return the natural logarithm of 1 + x | number | |
log10(x) | return the base 10 logarithm of x | number | |
log2(x) | return the base 2 logarithm of x | number | |
max(...args) | return the largest of the resulting values | number | |
min(...args) | return the smallest of the resulting values | number | |
pow(base, exponent) | return base x to the exponent power y (that is, x^y) | number | |
random() | return a pseudo-random number between 0 and 1 | number | |
round(x) | return the value of the number x rounded to the nearest integer | number | |
sign(x) | return the sign of the x, indicating whether x is positive, negative, or zero | number | |
sin(x) | return the sine of x | number | |
sinh(x) | return the hyperbolic sine of x | number | |
sqrt(x) | return the positive square root of x | number | |
tan(x) | return the tangent of x | number | |
tanh(x) | return the hyperbolic tangent of x | number | |
trunc(x) | return the integer portion of x, removing any fractional digits | number |
Note:
x ** y
is recommended to implement x ^ y instead ofMath.pow(x, y)
.~~x
is recommended to get the integer part of the given number instead ofMath.trunc(x)
.